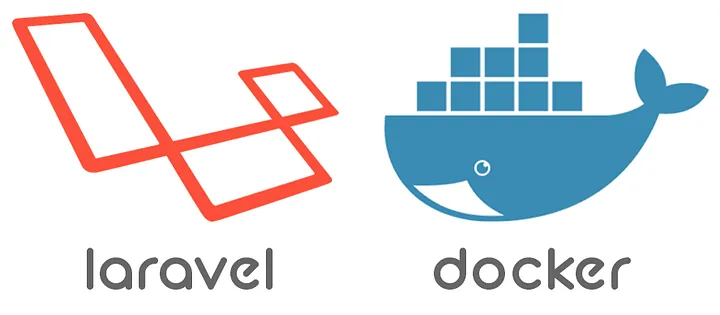
Docker has become a popular choice for developing and deploying applications due to its ease of use and portability. In this tutorial, we will guide you through the process of setting up a Laravel application with Docker, allowing you to quickly get started with Laravel development in a containerized environment.
Prerequisites
Before we begin, make sure you have Docker installed on your machine. Below are the steps of installing docker on different operating systems
Installing Docker on Linux
To install Docker on Linux, follow these steps
1) Update the package index on your system
sudo apt update
2) Install the required packages to allow apt to use a repository over HTTPS
sudo apt install -y apt-transport-https ca-certificates curl software-properties-common
3) Add the Docker GPG key to ensure the authenticity of the Docker packages
curl -fsSL https://download.docker.com/linux/ubuntu/gpg | sudo gpg --dearmor -o /usr/share/keyrings/docker-archive-keyring.gpg
4) Add the Docker repository to your system’s sources
echo "deb [arch=amd64 signed-by=/usr/share/keyrings/docker-archive-keyring.gpg] https://download.docker.com/linux/ubuntu $(lsb_release -cs) stable" | sudo tee /etc/apt/sources.list.d/docker.list > /dev/null
5) Update the package index again to include the Docker repository
sudo apt update
6) Install Docker
sudo apt install -y docker-ce docker-ce-cli containerd.io
7) Start the Docker service
sudo docker run hello-world
Installing Docker on macOS
To install Docker on macOS, follow these steps:
1) Download Docker Desktop for Mac from the Docker website (https://www.docker.com/) and double-click the downloaded .dmg
file to open the installer.
2) Drag the Docker.app file to the Applications folder.
3) Open Docker.app from the Applications folder.
4) Docker Desktop prompts you to authorize the installation with your system password. Enter your password and click “OK.”
5) Docker Desktop starts automatically after the installation is complete.
6) Docker Desktop shows a whale icon in the status bar at the top of your screen. It indicates that Docker is running and accessible from the terminal.
7) Open a terminal and verify that Docker is installed correctly by running a simple container
docker run hello-world
Installing Docker on Windows
To install Docker on Windows, follow these steps:
1) Download Docker Desktop for Windows from the Docker website (https://www.docker.com/) and run the installer.
2) Follow the prompts to install Docker Desktop.
3) After the installation is complete, Docker Desktop starts automatically.
4) Docker Desktop shows an icon in the system tray. It indicates that Docker is running and accessible from the command prompt or PowerShell.
5) Open Command Prompt or PowerShell and verify that Docker is installed correctly by running a simple container
docker run hello-world
By following these steps, you should be able to install Docker on Linux, macOS, and Windows successfully. Once Docker is installed, you can proceed with setting up your Laravel application with Docker as described in the previous sections.
If you encounter any issues during the installation process, refer to the Docker documentation or the specific documentation for your operating system for further troubleshooting steps.
Now let’s move on creating a Laravel Application. Sail is an alternative way to integrate Docker with Laravel. It provides a lightweight Docker-powered local development environment specifically tailored for Laravel applications.
Let’s go through both methods: using Docker directly and using Laravel Sail.
Method 1: Using Docker Directly
Step 1: Create a Laravel Application
To get started, we need to create a new Laravel project. Open your terminal or command prompt and navigate to the directory where you want to create your Laravel application. Run the following command to create a new Laravel project:
composer create-project --prefer-dist laravel/laravel your-project-name
Wait for the installation process to complete. This will create a new Laravel project in a directory named “your-project-name”.
Step 2: Dockerize Laravel Application
Now, let’s Dockerize our Laravel application. In the root directory of your Laravel project, create a new file called Dockerfile
. This file will define the Docker image for our Laravel application. Open the Dockerfile
and add the following content:
FROM php:7.4-fpm WORKDIR /var/www/html RUN apt-get update && apt-get install -y \ libzip-dev \ zip \ && docker-php-ext-configure zip \ && docker-php-ext-install zip RUN curl -sS https://getcomposer.org/installer | php -- --install-dir=/usr/local/bin --filename=composer COPY . . RUN composer install CMD php artisan serve --host=0.0.0.0 --port=8000
Let’s break down what each line does:
FROM php:7.4-fpm
: We base our image on the official PHP 7.4 FPM (FastCGI Process Manager) image.WORKDIR /var/www/html
: Sets the working directory within the container to/var/www/html
.RUN apt-get update && apt-get install -y ...
: Updates the package list inside the container and installs the necessary dependencies (in this case,libzip-dev
andzip
).RUN curl -sS https://getcomposer.org/installer | php -- --install-dir=/usr/local/bin --filename=composer
: Installs Composer inside the container.COPY . .
: Copies the contents of your current directory (the Laravel project files) into the container’s working directory.RUN composer install
: Runs the Composer install command to install the project dependencies.CMD php artisan serve --host=0.0.0.0 --port=8000
: Starts the Laravel development server when the container is run.
Save the Dockerfile
in the root directory of your Laravel project.
Step 3: Create an Environment File
APP_PORT=8000 DB_PASSWORD=your_db_password DB_DATABASE=your_db_name DB_USERNAME=your_db_user
Step 4: Create a Docker Compose File
Next, let’s create a Docker Compose file to define the services and their configurations. In the root directory of your Laravel project, create a new file called docker-compose.yml
. Open the docker-compose.yml
file and add the following content:
version: '3' services: app: build: context: . dockerfile: Dockerfile ports: - $APP_PORT:$APP_PORT volumes: - .:/var/www/html depends_on: - db env_file: - .env db: image: mysql:5.7 environment: MYSQL_ROOT_PASSWORD: $DB_PASSWORD MYSQL_DATABASE: $DB_DATABASE MYSQL_USER: $DB_USERNAME MYSQL_PASSWORD: $DB_PASSWORD phpmyadmin: image: phpmyadmin/phpmyadmin environment: PMA_HOST: db MYSQL_ROOT_PASSWORD: $DB_PASSWORD ports: - 8080:80 depends_on: - db env_file: - .env
In this docker-compose.yml
file, we define three services: app
, db
, and phpmyadmin
.
- The
app
service builds an image using the Dockerfile we created earlier. It maps port 8000 from the container to port 8000 on the host machine, allowing us to access the Laravel application. - The
db
service uses the official MySQL 5.7 image. We set environment variables for the MySQL root password, database name, user, and password. - The
phpmyadmin
service uses the phpMyAdmin image, which provides a web-based interface for managing MySQL databases. It is linked to thedb
service and maps port 8080 from the container to port 80 on the host machine.
Make sure to replace the placeholder values (your_password
, your_database
, your_user
) with your desired values.
Save the docker-compose.yml
file in the root directory of your Laravel project.
Step 5: Build and Run the Docker Containers
We’re now ready to build and run our Docker containers. Open your terminal or command prompt and navigate to the root directory of your Laravel project. Run the following command:
docker-compose up -d
This command will start building the Docker images and create the necessary containers based on the configurations defined in the docker-compose.yml
file. The -d
flag runs the containers in the background.
Wait for the process to complete. Once done, you should have your Laravel application running inside a Docker container.
Step 6: Access Your Laravel Application
Open your web browser and visit http://localhost:8000
. You should see your Laravel application up and running.
Additionally, you can access phpMyAdmin by visiting http://localhost:8080
. Log in using the MySQL root credentials you specified in the docker-compose.yml
file. From there, you can manage your MySQL databases using the phpMyAdmin web interface.
Method 2: Using Laravel Sail
Step 1: Install Laravel Sail
If you haven’t already, create a new Laravel project using the following command
composer create-project --prefer-dist laravel/laravel your-project-name
Once the project is created, navigate to the project directory:
cd your-project-name
Install Laravel Sail globally using Composer:
composer global require laravel/sail
Ensure that the Composer global bin directory is in your system’s $PATH
variable, so you can access the sail
command globally.
Step 2 : Generate Sail Configuration:
Run the following command to generate the Sail configuration files:
sail up
This will generate a docker-compose.yml
file and a Dockerfile
in the root of your Laravel project.
Step 3: Build and Run the Docker Containers
Run the following command to build and start the Docker containers defined in the Sail configuration
sail up -d
Laravel Sail offers additional features and services out of the box, such as a MySQL database container, Redis, and Mailhog for mail testing. You can customize the services and configurations in the docker-compose.yml
file generated by Sail to suit your needs.
Choose the method that suits your preferences and project requirements. Both methods provide Docker integration for your Laravel application, allowing you to develop and deploy in a containerized environment.
Conclusion
Congratulations! You have successfully set up a Laravel application with Docker. This containerized environment provides a convenient and reproducible setup for developing and deploying your Laravel projects. You can now start building your Laravel application within the Docker environment, utilizing the benefits of containerization.
Feel free to customize the Dockerfile and docker-compose.yml
file based on your project requirements. You can add more services, tweak configurations, or install additional dependencies to suit your needs.
Happy coding!
Comments are closed.