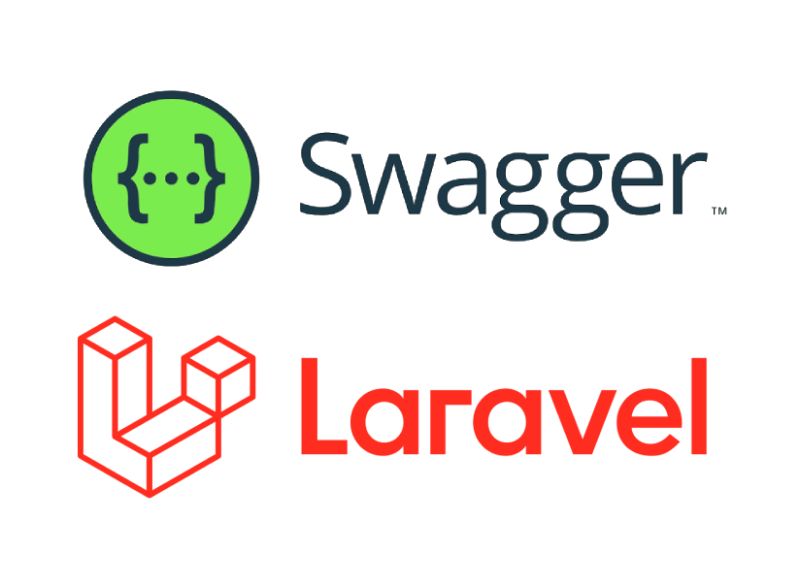
Laravel is a popular PHP framework, and Swagger is a powerful tool for designing, building, and documenting RESTful APIs. Integrating Laravel with Swagger can greatly enhance your API development process and make it easier to communicate and collaborate with other developers.
In this blog post, we will explore the steps involved in integrating Laravel with Swagger and discuss the benefits it offers. Let’s dive in!
What is Swagger?
Swagger is an open-source toolset that provides a set of tools for designing, building, documenting, and consuming RESTful APIs. It allows you to describe your API using a standard format called OpenAPI Specification (OAS), formerly known as Swagger Specification. The OAS defines a structured way to describe your API endpoints, request/response models, authentication mechanisms, and more.
Why integrate Laravel with Swagger?
Integrating Laravel with Swagger offers several advantages for API development:
1) API documentation
Swagger generates interactive API documentation automatically based on your API’s OpenAPI Specification. This documentation provides a clear and user-friendly interface for exploring your API’s endpoints, parameters, response formats, and even allows users to test API calls directly from the documentation.
2) Collaboration
With Swagger, you can share your API’s documentation with other developers, making it easier to collaborate on API development. Swagger provides a central location where developers can discuss API design, review endpoints, and suggest improvements, fostering better teamwork and communication.
3) Client SDK generation
Swagger can automatically generate client SDKs for various programming languages based on your API’s OpenAPI Specification. This makes it easier for developers to consume your API by providing them with pre-built code and client libraries tailored to their preferred programming language.
4) Validation and testing
Swagger allows you to validate your API endpoints against the defined OpenAPI Specification. This ensures that your API conforms to the expected structure, parameter types, and request/response formats. Additionally, Swagger provides built-in testing tools that allow you to test your API endpoints directly from the generated documentation.
Integrating Laravel with Swagger
To integrate Laravel with Swagger, you can follow these steps:
1) Install the necessary dependencies
Start by installing the darkaonline/l5-swagger
package in your Laravel project. This package provides seamless integration between Laravel and Swagger.
composer require "darkaonline/l5-swagger"
2) Configure the package
Once installed, you need to publish the package’s configuration file and customize it according to your project’s needs. The configuration file allows you to specify the location of your API’s OpenAPI Specification, customize the documentation appearance, and more.
php artisan vendor:publish --provider "L5Swagger\L5SwaggerServiceProvider"
3) Annotate your routes
To generate accurate documentation, you need to annotate your Laravel routes with Swagger annotations. These annotations provide additional information about each route, including the expected request/response formats, parameters, and authentication requirements.
In your Laravel routes file (typically routes/web.php
or routes/api.php
), you can annotate your routes using Swagger annotations. Here’s an example:
use L5Swagger\Http\Controllers\SwaggerController; Route::get('/user/{id}', [UserController::class, 'getUser']) ->name('users.show') ->middleware('auth') ->middleware('bindings') ->middleware('swagger.annotations') ->middleware('l5-swagger.responses:User');
4) Implement Swagger Annotations in Laravel Controller
use App\Http\Controllers\Controller; use Illuminate\Http\Request; use Swagger\Annotations as SWG; class UserController extends Controller { /** * Get a user by ID. * * @SWG\Get( * path="/user/{id}", * tags={"User"}, * summary="Get a user by ID", * @SWG\Parameter( * name="id", * in="path", * description="ID of the user", * required=true, * type="integer" * ), * @SWG\Response( * response=200, * description="User details" * ), * @SWG\Response( * response=404, * description="User not found" * ) * ) */ public function getUser($id) { // Logic to fetch the user by ID return response()->json($user); } // Additional methods and functionality can be implemented here }
4) Generate the OpenAPI Specification file
After annotating your routes, you can generate the OpenAPI Specification file by running a Laravel artisan command provided by the darkaonline/l5-swagger
package. This command scans your annotated routes and generates the OpenAPI Specification in JSON or YAML format.
php artisan l5-swagger:generate
5) View and test the documentation
Finally, you can access the Swagger documentation by visiting the specified URL in your browser. The generated documentation will present an interactive interface where you can explore your API’s endpoints, test API calls, and view the automatically generated client SDKs.
By following these steps, you can integrate Laravel with Swagger and leverage its powerful features to enhance your API development process.
At Eltech Systems, we have a team of experienced Laravel developers who are ready to provide top-notch development services for your business. With our deep understanding of the Laravel framework, we can create custom web applications, integrate APIs, develop Laravel packages, and offer ongoing support and maintenance.
Our developers follow best practices, deliver clean code, and prioritize effective communication throughout the development process. If you’re looking for Laravel expertise to take your project to the next level, don’t hesitate to get in touch with us. Contact us today to discuss your requirements and let our skilled team turn your ideas into reality.
Conclusion
In conclusion, integrating Laravel with Swagger offers numerous benefits for API development, including automatic API documentation generation, collaboration capabilities, client SDK generation, and validation/testing features. By combining the strengths of Laravel and Swagger, you can streamline your API development workflow and improve the overall quality and accessibility of your APIs.
Comments are closed.